How to Access YouTube Video Data using YouTube Data API and PHP?
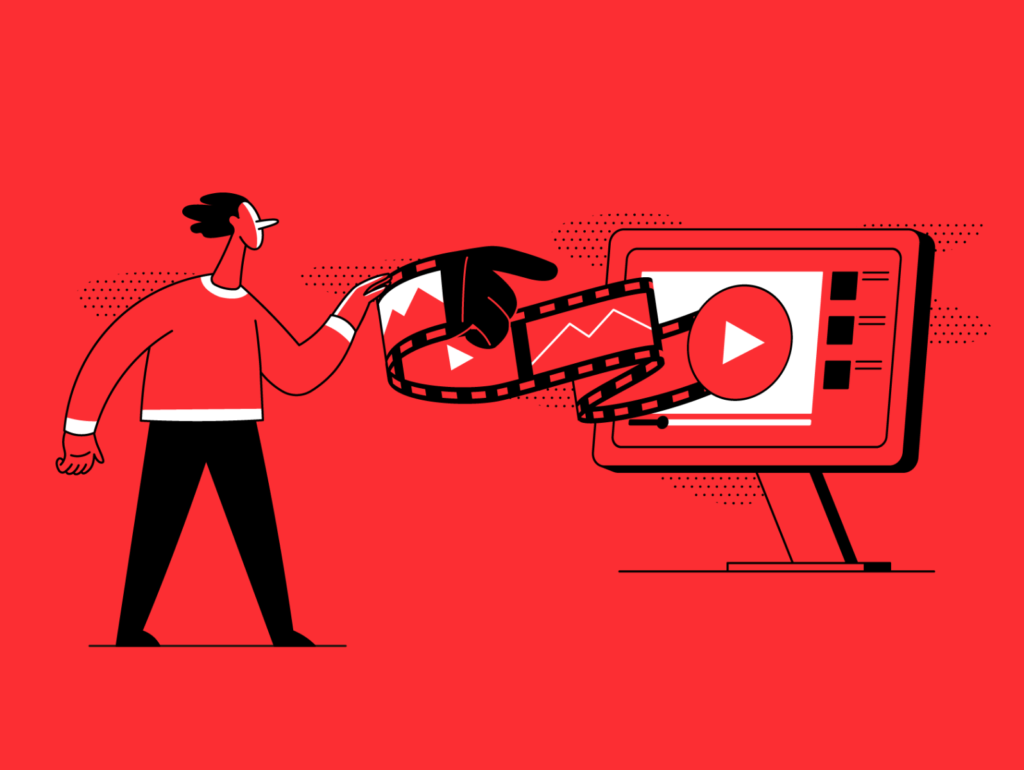
Today, who doesn’t know YouTube? It is a popular platform for sharing and watching videos online. While the platform is great for entertainment, it is equally purposeful for developers. Additionally, you will be surprised to know that you can access YouTube video data using YouTube data API and PHP.
YouTube Data API helps you to interact with other YouTube resources, including playlists, videos, channels, and more. Meanwhile, PHP, as a server-side scripting language, can be used to build dynamic websites, portals, and web apps. By integrating the power of both technologies, developers can easily access YouTube video data using the data API and PHP.
In this tutorial, we will demonstrate the step-wise process and sample code to access YouTube video data, such as title, description, likes, views, comments, and more. You will also learn to show the video data using HTML and CSS. So, let’s start now!
Step-by-Step Approach to Access YouTube Video Data Using YouTube API
Below are some steps to access the YouTube video data using the YouTube API and PHP code:
- Visit the Google Developer Console and log in to your existing Google account or create one.
- After signing in, click on the ‘Create Project’ button.
- Wait until the Google creates a developer’s project.
- Now, name the project as per your choice.
- Tap on the ‘Select a Project Link’ in the Google API console.
- Next, tap on the plus (+) button to create a new project.
- Enter the name of the project and click on the ‘Create’ button.
- Wait until Google sets up a new developer’s project. After that, choose the project.
- On the navigation menu, tap on the ‘Library’ link. Now, click on the ‘YouTube Data API’ button.
- Next, click on the ‘ENABLE’ button to enable the YouTube Data API v3.
- Now, tap the ‘Create Credentials’ button available on the left navigation menu.
- Following that, you will see a dialog box with the API Key that you just created. This is the key that you need to use in the API request for YouTube Data API v3.
Example Codes for Accessing YouTube Data Using YouTube Data API and PHP
HTML Code
The code below displays an HTML form that asks the user to enter a YouTube video URL. The URL value will be sent to the file “showDetails.php” when the user submits the form. This file will get the data from the URL and show it on the browser.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="style.css"> </head> <body> <br /> <p> Please enter the youtube URL in the input </p>
<div id="divID" class="container-class"> <form method="post" action="showDetails.php"> <input class="input-class" type="text" name="url" placeholder="Enter your URL"> <br /> <br /> <input class="submit-class" type="submit" name="submit" value="Submit URL"> </form> </div> </body> </html>
PHP Code
This code shows the file “showDetails.php” that is used by the HTML web page above.
<?php error_reporting (E_ALL ^ E_NOTICE); /*Just for your server-side code*/ header('Content-Type: text/html; charset=ISO-8859-1'); ?> <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content= "width=device-width, initial-scale=1"> <style> .thumbnail-class { width: 50%; margin: 10px; padding: 5px; border-radius: 1px; } #titleDescID { width: 50%; margin: 10px; padding: 10px; } </style> </head> <br /> <body> <div id="thumbnailID" class="thumbnail-class"> <?php if (isset($_POST['submit'])){ $url = $_POST['url']; /* Extracting the v element from the link*/ $vString = explode("v=", $url); $youtubeId = $vString[1]; } ?> <div id="videoDivID" style="width:600px;height:317px;"> <iframe id="iframe" style="width:100%;height:100%" src= "https://www.youtube.com/embed/<?php echo $youtubeId; ?>" data-autoplay-src=" https://www.youtube.com/embed/<?php echo $youtubeId; ?>?autoplay=1"> </iframe> </div> </div> <?php //Its different for all users $myApiKey = 'ENTER YOUR API KEY'; $googleApi = 'https://www.googleapis.com/youtube/v3/videos?id =' . $youtubeId . '&key=' . $myApiKey . '&part=snippet'; /* Create new resource */ $ch = curl_init(); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); /* Set the URL and options */ curl_setopt($ch, CURLOPT_URL, $googleApi); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_VERBOSE, 0); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); /* Grab the URL */ $curlResource = curl_exec($ch); /* Close the resource */ curl_close($ch); $youtubeData = json_decode($curlResource); $youtubeVals = json_decode( json_encode($youtubeData), true); $urlTitle = $youtubeVals ['items'][0]['snippet']['title']; $description = $youtubeVals ['items'][0]['snippet']['description']; ?> <div id="titleDescID"> <?php echo '<b>Title: ' . $urlTitle . '</b>'; echo '<b>Description: </b>' . $description; ?> </div> </body> </html>
CSS Code
The below-mentioned code shows the ‘style.css’ file that was used in the above HTML code.
body{ font-family: Sans-serif,Arial; width: 600px; } .container-class{ background: #e9e9e9; border: #B3B2B2 1px solid; border-radius: 2px; margin: 20px; padding: 40px; } .input-class{ width: 100%; border-radius: 2px; padding: 20px; border: #e9e9e9 1px solid; } .submit-class{ padding: 10px 20px; background: #000; color: #fff; font-size: 0.8em; width: 110px; border-radius: 4px; cursor:pointer; border: black 2px solid; }
Conclusion
In this tutorial, we have explained the process of accessing YouTube data using the YouTube Data API and PHP code. With the help of this guide, you can access the video data easily using the API.