Step-by-Step Tutorial for Passing Variables and Data from PHP to Javascript
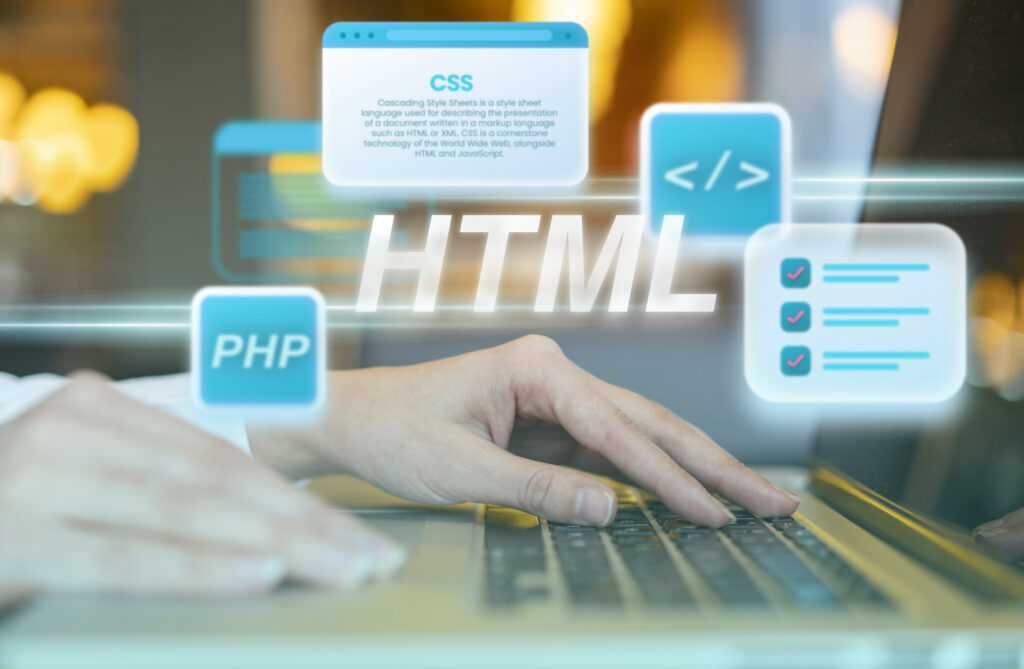
While working on web development projects, it is common for new developers to encounter situations where they may need to pass variables from PHP to Javascript, especially when they update a web page or manipulate data using Javascript on the client side. The good news is that it can be done easily and effectively in different ways. In this blog, we will discuss the five most common and effective methods to pass variables and data from PHP to Javascript.
Before diving into these methods, it is essential to understand why is it necessary to pass variables from PHP to JavaScript. As a server-side language, PHP executes on the server before a web page is delivered to the user. At the same time, JavaScript is a client-side language and doesn’t require execution.
By passing a variable from PHP to JavaScript, users can easily access the PHP data in their JavaScript code, which allows interactive and dynamic web pages.
5 Methods for Passing Variables and Data from PHP to JavaScript
Now that you have understood the importance of this integration, let’s explore the five effective methods you can use to pass variables from PHP to JavaScript.
Method 1: Inline PHP
The inline PHP method allows you to insert your PHP variable directly into your JavaScript code. For example, suppose you have a PHP variable named “$name” that has the value “alex”:
$name = "alex";
To use the PHP variable in your JavaScript code, you can directly insert its value using the PHP echo statement. For example:
<script> var jsName = ""; </script>
Here, we are creating a JavaScript variable named “jsName” and giving it the same value as the PHP variable “$name” by using the PHP echo statement inside the script tags. The final JavaScript code will look like this:
<script> var jsName = "alex"; </script>
You can now access the JavaScript variable “jsName” in your code that runs on the browser, knowing that it has the same value as the PHP variable “$name”. This technique lets you pass any PHP variable to JavaScript, such as strings, numbers, booleans, and even arrays.
Method 2: Using JSON
You can also use JSON (JavaScript Object Notation) to pass PHP variables to JavaScript. JSON is a convenient format for exchanging data between PHP and JavaScript.
To use JSON, you need to first turn your PHP variable into a string that follows the JSON syntax. PHP has a function called “json_encode()” that does this for you.
For example, we have a PHP array named “$person” that contains some information about a person:
$person = array( 'name' => 'alex', 'age' => 25, 'city' => 'New York' );
Using the ‘json_encode()’ function, we will now encode the array into JSON string:
$jsonPerson = json_encode($person); Now, the “$jsonPerson” variable have the JSON-encoded string: {"name":"alex","age":25,"city":"New York"}
We can then send this JSON string to JavaScript and convert it into a JavaScript object using the “JSON.parse()” function:
<script> var jsPerson = JSON.parse(''); </script>
As JSON offers a standardized and structured approach for passing data from PHP to JavaScript, it allows for easy and efficient data manipulation on the client side. However, JSOn may not work with other PHP resources like files or network sockets, database connections, etc.
Method 3: AJAX Request
Another powerful way to pass PHP variables to JavaScript is by using AJAX (Asynchronous JavaScript and XML) requests. AJAX lets you make HTTP requests to the server without reloading the whole web page, get data, and update your web page accordingly.
To use AJAX, you need to send a request to a PHP script that handles your data and sends back the result. You can do this using either the XMLHttpRequest or jQuery’s AJAX method.
Here’s an example using the native XMLHttpRequest:
<script> var xhr = new XMLHttpRequest(); xhr.open('GET', 'process.php', true); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { var data = JSON.parse(xhr.responseText); var name = data.name; // Use the 'name' variable in your JavaScript code } }; xhr.send(); </script>
This method enables more dynamic interactions between PHP and JavaScript, as you can send variables to the server, do server-side processing, and get the results back to JavaScript. However, it also requires extra server-side code to handle the AJAX request and process the data.
Method 4: Using Hidden Input Fields
You can alternatively use hidden input fields in your HTML form to pass PHP variables to JavaScript effectively. Hidden input fields are elements that store data that the user cannot see, but JavaScript can access and use.
To use hidden input fields, you need to create an input element with the “type” attribute set to “hidden” and the “value” attribute set to the PHP variable you want to pass. Here’s an example:
<input type="hidden" id="phpVariable" value="">
In this example, we will make a hidden input field with the ID “phpVariable” and assigning its value to the PHP variable “$myVariable”. The value will be printed directly into the HTML, making it available to JavaScript.
Then, you can get this hidden input field from JavaScript using its ID and get the value. Here’s an example:
<script> var jsVariable = document.getElementById("phpVariable").value; // Use the 'jsVariable' in your JavaScript code </script>
By using Javascript to retrieve the hidden input field value, you can use the PHP variable on client-side codes.
This method is helpful when you want to pass data from PHP to JavaScript within the context of an HTML form. It’s particularly useful when sending forms or doing client-side validation. However, it’s important to make sure that the hidden input field is properly protected and not altered on the client side, as users can change the value of hidden input fields.
Method 5: Passing Variables Directly from PHP in JavaScript Code
This method is suitable when you have a small piece of PHP code that you want to run within your JavaScript code block.
To pass PHP variables in JavaScript code, you can use the inline PHP tags (“) directly in your JavaScript code. Here’s an example:
<script> var phpVariable = ''; // Use the 'phpVariable' directly in your JavaScript code </script>
In this example, we’re setting the value of the PHP variable “$myVariable” to the JavaScript variable “phpVariable”. The PHP code within the inline PHP tags will be run on the server side, and the resulting value will be included in the JavaScript code when the page is loaded.
This method offers a simple way to pass PHP variables to JavaScript without the need for extra processing or server requests. It’s especially helpful when you have a small amount of data or want to do a specific action based on the PHP variable value within your JavaScript code.
Conclusion
Passing variables and data from PHP to JavaScript is a common task for web developers. In this article, we looked at five methods to do this: inline PHP, using JSON, AJAX requests, hidden input fields, and passing PHP variables directly in JavaScript code.
These methods have different advantages and use cases, and the choice depends on the specific requirements of your web development project. By learning these methods, you can pass PHP variables to JavaScript easily and effectively.