Step-by-Step Guide to Uploading an Image into a Database and Displaying it using PHP
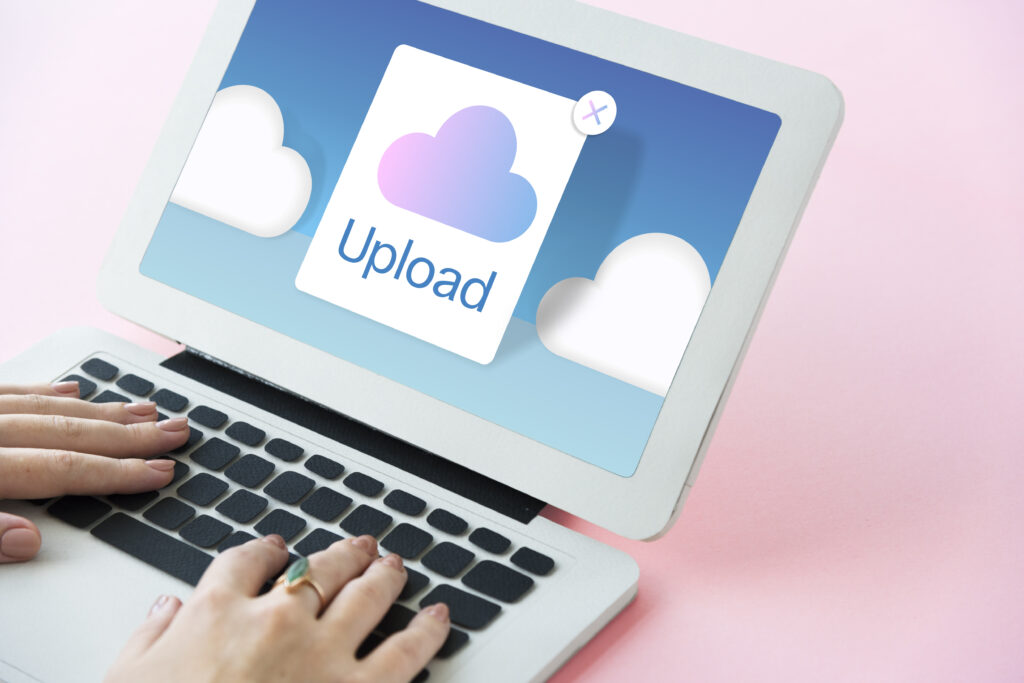
Cloud Computing Storage Icon Concept
Storing images in databases is an essential aspect of modern websites and web applications. You can store images on the database for various reasons such as easy management, security, and scalability. At the same time, PHP offers the easiest and safest way to upload an image to a database and display it on web pages. The best part is that you don’t need to write lengthy codes to upload your images to the database, just simply follow the steps and write the sample codes and you’re good to go.
Today, we will help you understand the process of uploading images into a database and displaying them on web pages using PHP through this step-by-step guide.
Below, we have demonstrated the entire process of image upload on the database with examples. The process will implement the file upload and display functionalities in the web application.
Prerequisites
Ensure you have a WAMP or XAMP server installed on your system to create a database table. Also, you can use tools such as phpMyAdmin to manage MySQL databases. So, let’s start the process of creating a database table using PHPMyAdmin.
Process to Upload Image into a Database and Display it using PHP
Step 1: Create a Database and Table
First, let’s start with creating a database with your own name. You can use any name for the database. Alternatively, you can use an existing database.
Following this step, create a table in the database to store the image data. The table should have a BLOB (Binary Large Object) column. These types of columns can store heavy binary data, including images and videos.
For instance, we have provided an SQL query for table creation with three columns: ID, Name, and Data. The name of the table will be “images.” The Id column will work as an auto-incremental key, the Name column will store the image’s filename, and the Data column will store the image’s binary data.
Use the following code to create a table in the SQL panel of PHPMyAdmin:
CREATE TABLE images ( id INT(11) NOT NULL AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, data LONGBLOB NOT NULL );
Step 2: Create an HTML Form for Image Uploading
After creating a table in the database, create an HTML form. It will enable users to choose and upload an image. The input field of the type of the form should be “file.” It will allow users to browse an image file from their computer and upload it. After the form submission, the selected file will be processed to the server.
<form method="post" action="upload.php" enctype="multipart/form-data"> <input type="file" name="image" /> <input type="submit" name="submit" value="Upload" /> </form>
Remember the HTML form has three crucial attributes:
- The ‘Method’ attribute, which is set to ‘Post’ specifies that the form data will be sent to the server through HTTP POST.
- The ‘Action’ attribute, which is set to ‘upload.php’ specifies that the PHP script name will handle the upload of the image file.
- The ‘Enctype’ attribute, which is set to ‘multipart/form-data’ is necessary for uploading image files.
Step 3: Processing the Uploaded Image
After creating an HTML form for uploading an image file, the next step is to create a PHP script. It will process and store the uploaded image in the database. However, you will need to ensure that the image file has been uploaded. If it is, extract the file’s binary data and store it in the database.
Here’s an example PHP script – upload.php:
<?php // check if an image file was uploaded if(isset($_FILES['image']) && $_FILES['image']['error'] == 0) { $name = $_FILES['image']['name']; $type = $_FILES['image']['type']; $data = file_get_contents($_FILES['image']['tmp_name']); // connect to the database $pdo = new PDO('mysql:host=localhost;dbname=mydb', 'username', 'password'); // insert the image data into the database $stmt = $pdo->prepare("INSERT INTO images (name, type, data) VALUES (?, ?, ?)"); $stmt->bindParam(1, $name); $stmt->bindParam(2, $type); $stmt->bindParam(3, $data); $stmt->execute(); } ?>
Step 4: Create a PHP Script to Display the Image
The last step is to create a PHP script to retrieve the data of the image from the database. The image will then be displayed on a web page. Using the SELECT statement, you must first retrieve the image data from the database. Now, using the MIME type, display the image data as binary data.
Here’s an example PHP script – display.php:
<?php // get the ID of the image from the URL $id = $_GET['id']; // connect to the database $pdo = new PDO('mysql:host=localhost;dbname=mydb', 'username', 'password'); // retrieve the image data from the database $stmt = $pdo->prepare("SELECT name, type, data FROM images WHERE id=?"); $stmt->bindParam(1, $id); $stmt->execute(); // set the content type header header("Content-Type: image/jpeg"); // output the image data $row = $stmt->fetch(PDO::FETCH_ASSOC); echo $row['data']; ?>
Step 5: Display the Image on a Web Page
Use the HTML ‘img’ tag to display the image on the web page. Now, set the ‘src’ attribute to the PHP script URL to output the image data.
Here’s an example HTML code displaying an image with ID 1:
<img src="display.php?id=1" />
When you load the page, the ‘display.php’ script will run with the ID parameter set to 1. The image will be displayed as binary data with ‘img’ tag.
Conclusion
The process of uploading an image to a database and displaying it on a web page is quite simple and involves following some easy steps. You will need to create a database table with BLOB columns, then an HTML form for image upload, a PHP script to process the image uploaded, and retrieve its data from the database.
Following these steps, your image will be displayed as binary data on the web page. You can follow our guide to get started with uploading image files to the database and displaying them on a web page.