A Beginner’s Guide to Email Retrieval Using PHP and IMAP
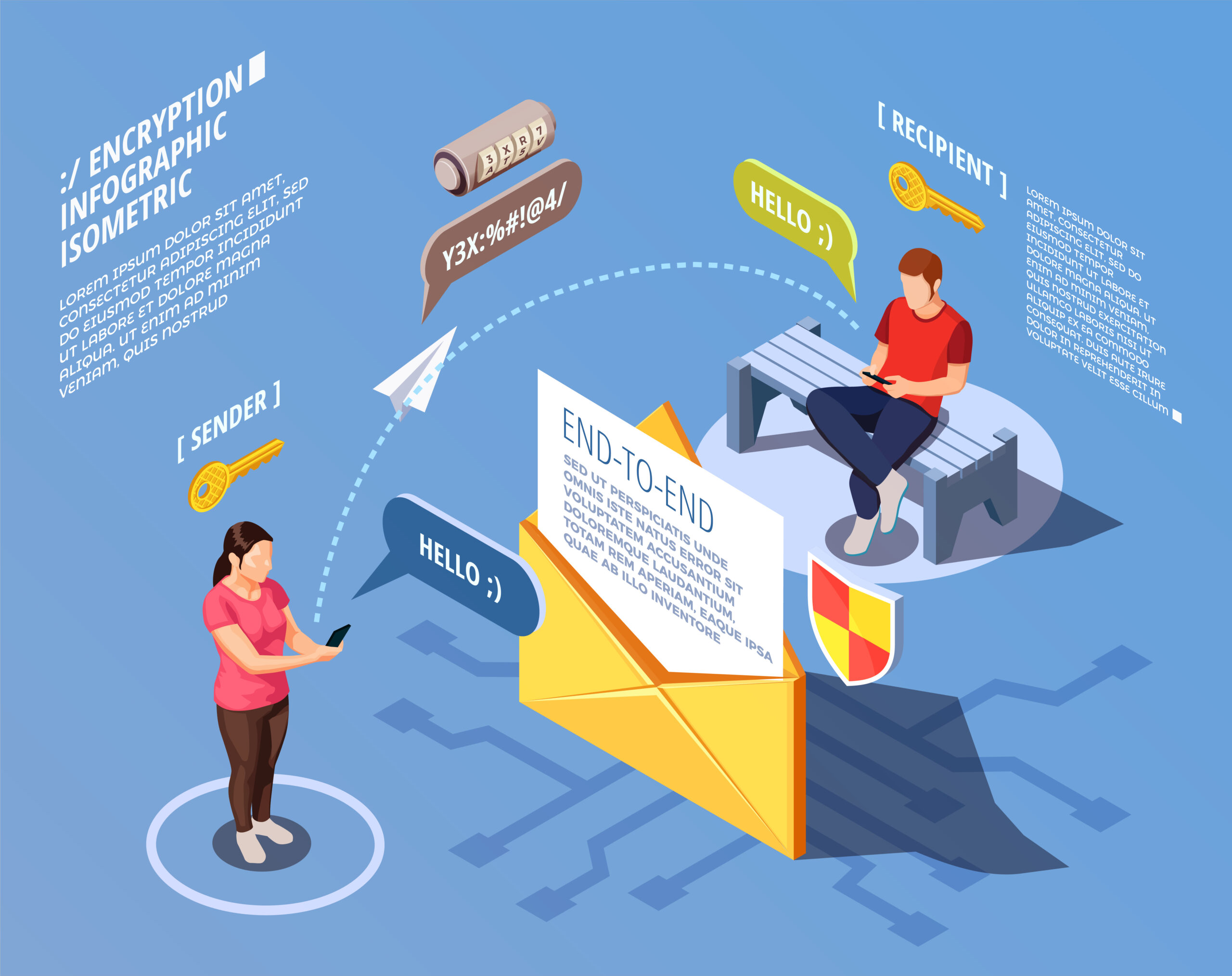
Data encryption cyber security isometric composition with encrypted messaging infographic icons and human characters of keypals vector illustration
Getting emails from Gmail accounts and reading them using PHP is probably the most enriching task for web developers. The simplicity of the PHP code through IMAP (Internet Message Access Protocol) is what makes the process more exciting. As a web development company, we often get a requirement from a client who needs email retrieval using PHP 8.
The email retrieval feature in PHP and its IMAP extension is particularly useful in email newsletters or email marketing strategies that send emails to customers automatically on a particular schedule.
IMAP is basically an ISP (Internet Standard Protocol) for users to retrieve emails from the server over a TCP/IP connection. The IMAP extension in PHP allows for effective email structure processing and offers access to email inboxes through communication with the email servers.
In this write-up, we will connect the Gmail server using PHP code and use the IMAP function to get and fetch emails from Gmail based on certain criteria. So, let’s start with the basic requirements for email retrieval using PHP. For the development of such functionality, we need the following:
- PHP5 or the latest PHP version.
- IMAP should be enabled in Gmail account settings.
- Enable the IMAP Extension in PHP installation.
Steps for Enabling Email Retrieval Using PHP and IMAP
Let’s move forward to understand the step-wide process of enabling IMAP in XAMPP and Gmail accounts to get emails.
Steps for Enabling IMAP in XAMPP
- To enable the IMAP extension in PHP, you need to modify the php.ini configuration file.
- Browse “;extension=php_imap.dll” and remove the semicolon at the beginning.
- It should look like this: “extension=php_imap.dll”.
- You also need to change the value of max_execution_time to 4000. This will allow PHP to run longer scripts without timing out.
Steps for Enabling IMAP in Gmail Account
To activate the IMAP extension in Gmail, you need to follow these steps:
- Open your Gmail account.
- Click on the Settings icon.
- Choose the Forwarding and POP/IMAP blue tab.
- Go to the “IMAP Access:” section and select the Enable IMAP radio button.
- Click on Save Changes.
- Make sure to turn on access for less secure apps for your Gmail account.
Note: The IMAP server usually listens on port number 143 for normal applications for email retrieval using PHP.
PHP Code for Email Retrieval using IMAP
The code below explains the process of email retrieval using PHP and IMAP. It shows how to display a list of emails from a Gmail account using HTML and PHP. The code requires the user’s “username” and “password” to be specified in order to connect to Gmail. After the connection is established, the code uses the imap_search() function to find all the emails or only the ones that match certain criteria.
The code then sorts the emails in reverse order using the PHP rsort() function, so that the most recent ones appear first. For each email, the code extracts the subject, sender, partial content, and date-time information.
The code uses the imap_fetchbody() function to get a specific part of the email body. To get the plain text part of the email, the code passes “1.1” as the third argument to this function. Here’s a sample code for email retrieval using PHP and IMAP:
HTML Code
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href= "https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css"> <link rel="stylesheet" href="style.css"> <script> function getEmails() { document.getElementById('dataDivID') .style.display = "block"; } </script> </head> <body> <h2>List Emails from Gmail using PHP and IMAP</h2> <div id="btnContainer"> <button class="btn active" onclick="getEmails()"> <i class="fa fa-bars"></i>Click to get gmail mails </button> </div> <br> <div id="dataDivID" class="form-container" style="display:none;"> <?php /* gmail connection,with port number 993 */ $host = '{imap.gmail.com:993/imap/ssl/ novalidate-cert/norsh}INBOX'; /* Your gmail credentials */ $user = 'YOUR-EMAIL@GMAIL.COM'; $password = 'YOUR-PASSWORD'; /* Establish a IMAP connection */ $conn = imap_open($host, $user, $password) or die('unable to connect Gmail: ' . imap_last_error()); /* Search emails from gmail inbox*/ $mails = imap_search($conn, 'SUBJECT "Comment"'); /* loop through each email id mails are available. */ if ($mails) { /* Mail output variable starts*/ $mailOutput = ''; $mailOutput.= '<table><tr><th>Subject </th><th> From </th> <th> Date Time </th> <th> Content </th></tr>'; /* rsort is used to display the latest emails on top */ rsort($mails); /* For each email */ foreach ($mails as $email_number) { /* Retrieve specific email information*/ $headers = imap_fetch_overview($conn, $email_number, 0); /* Returns a particular section of the body*/ $message = imap_fetchbody($conn, $email_number, '1'); $subMessage = substr($message, 0, 150); $finalMessage = trim(quoted_printable_decode($subMessage)); $mailOutput.= '<div class="row">'; /* Gmail MAILS header information */ $mailOutput.= '<td><span class="columnClass">' . $headers[0]->subject . '</span></td> '; $mailOutput.= '<td><span class="columnClass">' . $headers[0]->from . '</span></td>'; $mailOutput.= '<td><span class="columnClass">' . $headers[0]->date . '</span></td>'; $mailOutput.= '</div>'; /* Mail body is returned */ $mailOutput.= '<td><span class="column">' . $finalMessage . '</span></td></tr></div>'; }// End foreach $mailOutput.= '</table>'; echo $mailOutput; }//endif /* imap connection is closed */ imap_close($conn); ?> </div> </body> </html>
CSS Code
body { font-family: Arial; } table { font-family: arial, sans-serif; border-collapse: collapse; width: 100%; } tr:nth-child(even) { background-color: #dddddd; } td, th { padding: 8px; width:100px; border: 1px solid #dddddd; text-align: left; } .form-container { padding: 20px; background: #F0F0F0; border: #e0dfdf 1px solid; border-radius: 2px; } * { box-sizing: border-box; } .columnClass { float: left; padding: 10px; } .row:after { content: ""; display: table; clear: both; } .btn { background: #333; border: #1d1d1d 1px solid; color: #f0f0f0; font-size: 0.9em; width: 200px; border-radius: 2px; background-color: #f1f1f1; cursor: pointer; } .btn:hover { background-color: #ddd; } .btn.active { background-color: #666; color: white; }
Conclusion
Email Retrieval using PHP and IMAP is a great developer’s skill. Using both technologies together, web developers can create a code that connects Gmail and other email service providers, sort them in reverse order, and display all the details. So, that’s it in this guide to email retrieval using PHP and IMAP. For more details, you can contact us and our experts would be happy to assist you.
FAQs
What is IMAP used for email fetching?
IMAP, or Internet Message Access Protocol, is used for fetching emails from a mail server. It allows users to view and manage their emails directly on the server, providing real-time access from multiple devices. IMAP maintains email status (read, unread, flagged) across all devices and allows for more advanced email management compared to POP3. It’s particularly useful for users who access their email from various locations and devices.
How to use IMAP in PHP?
To use IMAP in PHP, you need to enable the IMAP extension in your PHP installation. Then, you can use PHP’s imap_open()
function to connect to an IMAP server with your email credentials. Once connected, use functions like imap_search()
to find emails, imap_fetch_overview()
to retrieve email summaries, and imap_body()
to read email content. Always ensure to close the connection with imap_close()
after completing your operations.
How to send an email in PHP step by step?
Sending an email in PHP typically involves using the mail()
function. First, define the recipient’s email address, subject, and message body. Optionally, set headers for additional details like “From” and “Reply-To”. Then, call the mail()
function with these parameters. For more robust email sending, use libraries like PHPMailer, which support attachments, HTML content, and SMTP authentication, providing greater control and security for sending emails.
What is email retrieval?
Email retrieval refers to the process of accessing and fetching emails from a mail server to a local client or application. This can be done using protocols like IMAP or POP3. IMAP retrieves email while keeping it on the server, allowing for synchronization across multiple devices. POP3, on the other hand, typically downloads and removes the email from the server, storing it locally. Retrieval ensures users can read, organize, and manage their email correspondence efficiently.
How to Do Email Retrieval Using PHP and IMAP?
To do email retrieval using PHP and IMAP, start by ensuring the IMAP extension is enabled in your PHP configuration. Next, use the imap_open()
function to connect to the IMAP server with your email credentials. You can then use imap_search()
to find specific emails based on criteria like ‘ALL’, ‘UNSEEN’, or ‘FROM’. Once you have the email IDs, retrieve details using imap_fetch_overview()
and get the email body with imap_fetchbody()
. Finally, close the connection with imap_close()
to ensure resources are freed up.